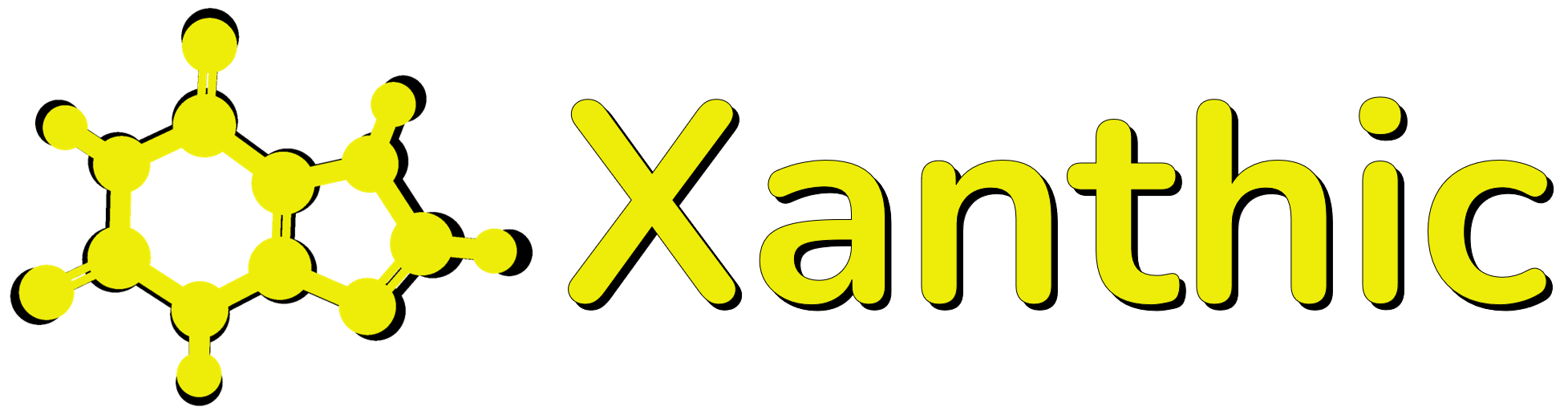
Xanthic is a straightforward API for in-memory caching on the JVM, via the facade pattern.
- Library developers can utilize Xanthic to enable caching without marrying a single implementation
- Application developers can utilize Xanthic to easily switch between different backing implementations whenever desired
Usage Example
Create Cache
Create a cache (with String
keys and Integer
values)
that can hold up to 2048 elements,
where entries expire 5 minutes following their latest access,
and removed entries notify a specific event listener:
- Java
- Kotlin
Cache<String, Integer> cache = CacheApi.create(spec -> {
spec.maxSize(2048L);
spec.expiryTime(Duration.ofMinutes(5L));
spec.expiryType(ExpiryType.POST_ACCESS); // or ExpiryType.POST_WRITE
spec.removalListener((key, value, cause) -> {
if (cause.isEviction()) {
// do something
}
});
});
val cache = createCache<String, Int> {
maxSize = 2048
expiryTime = Duration.ofMinutes(5)
expiryType = ExpiryType.POST_ACCESS // or ExpiryType.POST_WRITE
removalListener { key, value, cause ->
if (cause.isEviction) {
// do something
}
}
}
Since CacheApiSpec#provider(CacheProvider)
was not specified, the default cache provider will be used.
Default Cache Provider
- Application developers should add at least one
cache-provider-𝑥
module to their project (or create their own) - When there is only one cache provider registered, it will automatically become the default cache provider
- When there are multiple cache provider modules installed, the default will be selected according to the discovery order (but it is not recommended to rely on this order)
- Users can explicitly define which cache provider should be used as the default by calling CacheApiSettings. This will override whatever default was chosen from the discovery order